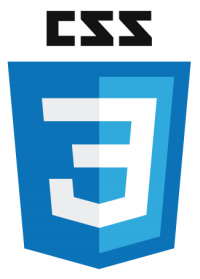
I’ve recently had to update the code that’s responsible for pulling in my latest YouTube upload on the front page. I had previously used a solution that uses the channel name rather than the channel ID. Apparently that API has stopped working and I had to find a workaround.
In addition to making it work again, I also wanted my embed to be responsive and look handsome on all screen sizes. The trouble is that regular iFrame embeds use a static size for the video (like 600×360 or 800×480), but being the future-proof thinking kind of guy that I am, I wondered if there was a way to make this dynamic. The “height=auto” trick didn’t work for me, but thankfully I came across a great piece of CSS that adds this feature.
Let me show you both parts to solving this puzzle.
Pulling the video from YouTube
In WordPress, a single video or playlist can easily be embedded by adding the URL on a new line in the post. The heavy lifting to build the iFrame is done under the hood. Sadly we need to do this manually with a little JavaScript. Here it is:
Take a look at the second line referencing a variable called cid. Replace that with your own channel ID. Speaking of variables, the vnum parameter is responsible for which of your uploads will be shown. 0 is the latest, 1 would be the previous one, 2 the one prior to that, and so forth.
Many thanks to Codegena for making this code available, and documenting it so well too! The only bit I’ve changed here is to add the rel=0 parameter so that related video won’t be shown at the bottom (in line 16). I’ve also enclosed this in a container of its own, which we’ll talk about next.
Note that the static size of this iFrame is 800×480. It’ll work as is, but the fascinating bit comes next: making it resize responsively.
Adding responsive CSS to our iFrame
Ben Marshall has a superb article about the ins and outs of responsive iFrames. To make this work for the above code, I’ll write two pieces of CSS: one for the video itself, and another for a container on the outside.
/* YouTube embed */ .latestVideoEmbed { border: 0; height: 100%; left: 0; position: absolute; top: 0; width: 100%; } /* responsive container */ .iframe-container { overflow: hidden; /* 16:9 aspect ratio */ padding-top: 56.25%; position: relative; }The first block sets the width and height of the video inside our container. It’s overriding the static size we’ve given it previously. The second block makes sure the aspect ratio is retained so that videos are not cropped. When the container resizes due to a new browser width, the video resizes with it.
Try it out with the video below, I’ve used this code as a demo with the latest video from my own YouTube channel.